Savoy ActiveX Control
|
This tutorial will explain programming with Savoy by making a mini host application.
|
Specification of Mini Host
Following is the specification of mini host which will control a general wafer inspection tool.
|
Create New Project
-
Launch Visual Studio 2017 and click [New] - [Project...] from File menu.
-
Choose "Windows Forms Application" from "Visual C#" project type, and type project name and folder name. For example, project name could be "SavoyTutorialCS2008". If setting was OK, click "OK" button.
-
New project was created.
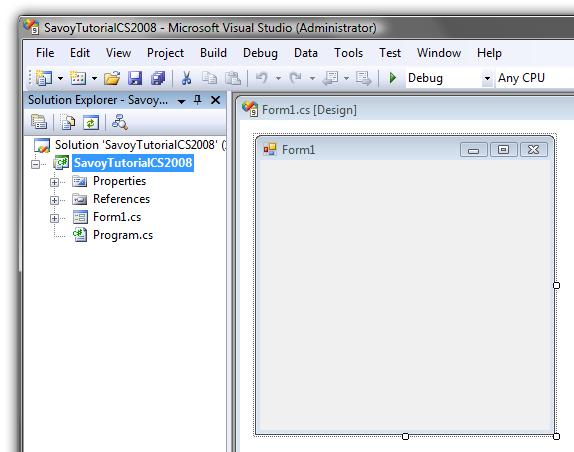
|
Add Savoy into Toolbox
|
Paste Savoy into Form
-
Place 1 SavoyHsms and 2 SavoySecsIIs on the form as follows.
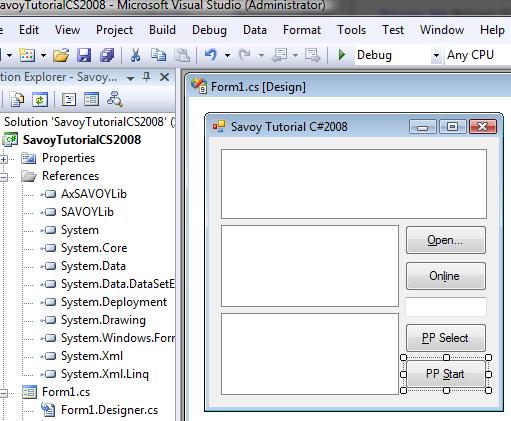
-
Make button event handler function.
If user clicks "Open" button, communication setting dialog box of SavoyHsms will appear on the screen.
And then establish connection, if user clicks "Open" button.
Since communication setting would be saved in "Savoy.ini" file, user doesn't have to change the setting next time.
|
private void button1_Click(object sender, EventArgs e)
{
// Setup
hsms.LoadIniFile();
if (hsms.Setup(""))
{
// If OK button was pressed, establish connection
hsms.Connect = true;
}
}
|
-
If user clicks "Online" button, send S1F13 message.
|
private void button2_Click(object sender, EventArgs e)
{
// Send S1F13
outmsg.SML = "s1f13w{}";
hsms.Send(outmsg.Msg);
}
|
-
If user clicks "PP Select" button, send remote command "PP-SELECT".
|
private void button3_Click(object sender, EventArgs e)
{
// Send S2F41 PP-Select
outmsg.SML = "s2f41w{<a'PP-SELECT'>{{<a'PPID'><a'" + textBox1.Text + "'>}}}";
hsms.Send(outmsg.Msg);
}
|
-
If user clicks "PP Start" button, send remote command "START".
|
private void button4_Click(object sender, EventArgs e)
{
// Send S2F41 Start
outmsg.SML = "s2f41w{<a'START'>{{}}}";
hsms.Send(outmsg.Msg);
}
|
|
Event Procedure
Capture events from SavoyHsms control.
-
If Connected event occurs, send select request message.
|
private void hsms_Connected(object sender, AxSAVOYLib._DSavoyHsmsEvents_ConnectedEvent e)
{
// Connected
// Send select request
outmsg.SML = "Select.req";
hsms.Send(outmsg.Msg);
}
|
-
If Received event occurs, pass incoming message to SavoySecsII control to analyze message structure.
|
private void hsms_Received(object sender, AxSAVOYLib._DSavoyHsmsEvents_ReceivedEvent e)
{
inmsg.Msg = e.lpszMsg;
|
-
If incoming message requires reply message, return appropriate message such as "<b 0>".
|
switch(inmsg.SType)
{
case 0:
// Data message
if(inmsg.Wbit && (inmsg.Function % 2)!=0)
{
// Need to reply something...
outmsg.SML = "<b 0>";
outmsg.Reply(e.lpszMsg);
hsms.Send(outmsg.Msg);
}
break;
|
-
If select request message arrives, reply select response message.
|
case 1:
// Select request
outmsg.SML = "Select.rsp";
outmsg.Reply(e.lpszMsg);
hsms.Send(outmsg.Msg);
break;
|
|
Entire Source Code
That's it.
This project was created from zero, however, the number of lines of entire source code is only 83 lines including empty lines and comments.
Actual code we wrote was only 30 lines except comment lines.
We didn't write any configuration file or data file which typically was required by competitors' products.
Competitors' products are never as simple as Savoy.
|
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace SavoyTutorialCS2008
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// Setup
hsms.LoadIniFile();
if (hsms.Setup(""))
{
// If OK button was pressed, establish connection
hsms.Connect = true;
}
}
private void button2_Click(object sender, EventArgs e)
{
// Send S1F13
outmsg.SML = "s1f13w{}";
hsms.Send(outmsg.Msg);
}
private void button3_Click(object sender, EventArgs e)
{
// Send S2F41 PP-Select
outmsg.SML = "s2f41w{<a'PP-SELECT'>{{<a'PPID'><a'" + textBox1.Text + "'>}}}";
hsms.Send(outmsg.Msg);
}
private void button4_Click(object sender, EventArgs e)
{
// Send S2F41 Start
outmsg.SML = "s2f41w{<a'START'>{{}}}";
hsms.Send(outmsg.Msg);
}
private void hsms_Connected(object sender, AxSAVOYLib._DSavoyHsmsEvents_ConnectedEvent e)
{
// Connected
// Send select request
outmsg.SML = "Select.req";
hsms.Send(outmsg.Msg);
}
private void hsms_Received(object sender, AxSAVOYLib._DSavoyHsmsEvents_ReceivedEvent e)
{
inmsg.Msg = e.lpszMsg;
switch(inmsg.SType)
{
case 0:
// Data message
if(inmsg.Wbit && (inmsg.Function % 2)!=0)
{
// Need to reply something...
outmsg.SML = "<b 0>";
outmsg.Reply(e.lpszMsg);
hsms.Send(outmsg.Msg);
}
break;
case 1:
// Select request
outmsg.SML = "Select.rsp";
outmsg.Reply(e.lpszMsg);
hsms.Send(outmsg.Msg);
break;
}
}
}
}
|
|
|