Savoy ActiveX Control
|
This tutorial will explain programming with Savoy by making a mini host application.
|
Specification of Mini Host
Following is the specification of mini host which will control a general wafer inspection tool.
|
Create New Project
-
Launch Visual Studio 2017 and click [New] - [Project...] from File menu.
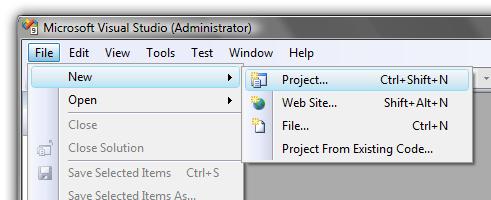
-
Choose "Windows Forms Application" from "Visual Basic" project type, and type project name and folder name.
For example, project name could be "SavoyTutorialVB2008".
If setting was OK, click "OK" button.
-
New project was created.
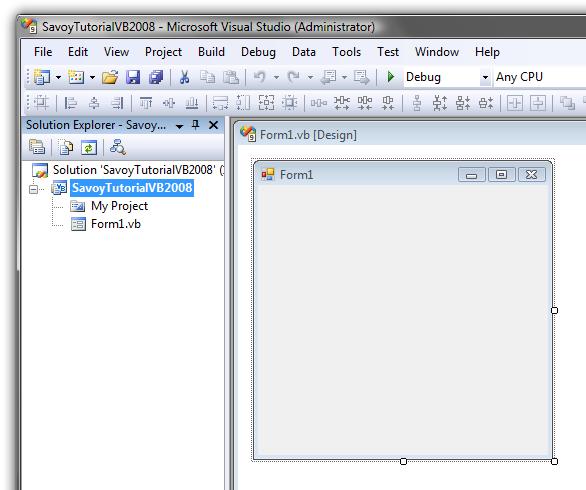
|
Add Savoy into Toolbox
|
Paste Savoy into Form
-
Place 1 SavoyHsms and 2 SavoySecsIIs on the form as follows.
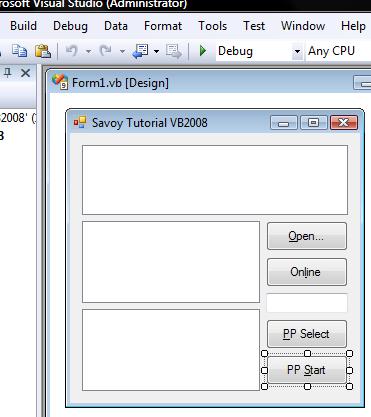
-
Make button event handler function.
If user clicks "Open" button, communication setting dialog box of SavoyHsms will appear on the screen.
And then establish connection, if user clicks "Open" button.
Since communication setting would be saved in "Savoy.ini" file, user doesn't have to change the setting next time.
|
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
' Setup
hsms.LoadIniFile()
If hsms.Setup("") Then
' If OK button was pressed, establish connection
hsms.Connect = True
End If
End Sub
|
-
If user clicks "Online" button, send S1F13 message.
|
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
' Send S1F13
outmsg.SML = "s1f13w{}"
hsms.Send(outmsg.Msg)
End Sub
|
-
If user clicks "PP Select" button, send remote command "PP-SELECT".
|
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
' Send S2F41 PP-Select
outmsg.SML = "s2f41w{<a'PP-SELECT'>{{<a'PPID'><a'" + TextBox1.Text + "'>}}}"
hsms.Send(outmsg.Msg)
End Sub
|
-
If user clicks "PP Start" button, send remote command "START".
|
Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
' Send S2F41 Start
outmsg.SML = "s2f41w{<a'START'>{{}}}"
hsms.Send(outmsg.Msg)
End Sub
|
|
Event Procedure
Capture events from SavoyHsms control.
-
If Connected event occurs, send select request message.
|
Private Sub hsms_Connected(ByVal sender As System.Object, ByVal e As AxSAVOYLib._DSavoyHsmsEvents_ConnectedEvent) Handles hsms.Connected
' Connected
' Send select request
outmsg.SML = "Select.req"
hsms.Send(outmsg.Msg)
End Sub
|
-
If Received event occurs, pass incoming message to SavoySecsII control to analyze message structure.
|
Private Sub hsms_Received(ByVal sender As System.Object, ByVal e As AxSAVOYLib._DSavoyHsmsEvents_ReceivedEvent) Handles hsms.Received
inmsg.Msg = e.lpszMsg
|
-
If incoming message requires reply message, return appropriate message such as "<b 0>".
|
Select Case inmsg.SType
Case 0
' Data message
If inmsg.Wbit And (inmsg.Function Mod 2) <> 0 Then
' Need to reply something...
outmsg.SML = "<b 0>"
outmsg.Reply(e.lpszMsg)
hsms.Send(outmsg.Msg)
End If
|
-
If select request message arrives, reply select response message.
|
Case 1
' Select request
outmsg.SML = "Select.rsp"
outmsg.Reply(e.lpszMsg)
hsms.Send(outmsg.Msg)
|
|
Entire Source Code
That's it.
This project was created from zero, however, the number of lines of entire source code is only 55 lines including empty lines and comments.
Actual code we wrote was only 25 lines except comment lines.
We didn't write any configuration file or data file which typically was required by competitors' products.
Competitors' products are never as simple as Savoy.
|
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
' Setup
hsms.LoadIniFile()
If hsms.Setup("") Then
' If OK button was pressed, establish connection
hsms.Connect = True
End If
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
' Send S1F13
outmsg.SML = "s1f13w{}"
hsms.Send(outmsg.Msg)
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
' Send S2F41 PP-Select
outmsg.SML = "s2f41w{<a'PP-SELECT'>{{<a'PPID'><a'" + TextBox1.Text + "'>}}}"
hsms.Send(outmsg.Msg)
End Sub
Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
' Send S2F41 Start
outmsg.SML = "s2f41w{<a'START'>{{}}}"
hsms.Send(outmsg.Msg)
End Sub
Private Sub hsms_Connected(ByVal sender As System.Object, ByVal e As AxSAVOYLib._DSavoyHsmsEvents_ConnectedEvent) Handles hsms.Connected
' Connected
' Send select request
outmsg.SML = "Select.req"
hsms.Send(outmsg.Msg)
End Sub
Private Sub hsms_Received(ByVal sender As System.Object, ByVal e As AxSAVOYLib._DSavoyHsmsEvents_ReceivedEvent) Handles hsms.Received
inmsg.Msg = e.lpszMsg
Select Case inmsg.SType
Case 0
' Data message
If inmsg.Wbit And (inmsg.Function Mod 2) <> 0 Then
' Need to reply something...
outmsg.SML = "<b 0>"
outmsg.Reply(e.lpszMsg)
hsms.Send(outmsg.Msg)
End If
Case 1
' Select request
outmsg.SML = "Select.rsp"
outmsg.Reply(e.lpszMsg)
hsms.Send(outmsg.Msg)
End Select
End Sub
End Class
|
|
|