Swing ActiveXコントロール
|
この章ではHSMSのミニクライアントを作成しながらプログラミングについて解説していくことにします。開発する言語はVisual Basicを使用します。
|
準備
最初にミニホストの時と同じように、新規プロジェクトを作成し、Swing ActiveXコントロールを挿入しておきます。
|
HSMSクライアントを作ってみよう
-
ActiveXコントロールを貼り付ける
SwingHsmsコントロールとSwingSecsIIコントロールをフォームに貼り付けます。今回は説明を簡単にするためにSwingSecsIIコントロールは一個だけ貼り付けます。
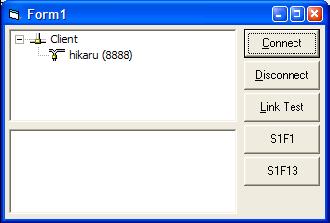
-
サーバと接続してみる
アプリケーションが起動した直後に接続を有効にするために、FormLoadイベントに以下のように追加してやります。
'接続する
.Active = True
-
送信の準備
HSMSのメッセージヘッダはSECS-Iとは異なります。このため、送信する前に専用の送信関数を作成して、若干の修正を加えてやる必要があります。
Private Sub Send (bDataMessage As Boolean)
With SwingSecsII1
If bDataMessage Then
.SessionID = 0
.SType = 0
Else
.SessionID = &HFFFF
End If
.PType = 0
.EBit = False
SwingHsms.Send .Msg
End With
End Sub
-
セレクト要求を送信する
HSMS-SS(HSMSシングルセッション)の場合はクライアント(アクティブエンティティ)側が'Select Request'(セレクト要求)をサーバ(パッシブエンティティ)に送る必要があります。
'接続に成功したか?
If .Active Then
'Select.Req送信
SwingSecsII1.List = "SelectReq"
Send False
End If
-
HSMSメッセージの受信
HSMSで定義されているメッセージのタイプは以下のものがあります。
0 | データメッセージ |
1 | セレクト要求 |
2 | セレクト応答 |
3 | セレクト解除要求 |
4 | セレクト解除応答 |
5 | リンクテスト要求 |
6 | リンクテスト応答 |
7 | リジェクト要求 |
9 | セパレート要求 |
Readイベントでは毎回受信したメッセージをチェックしてやる必要があります。
With SwingSecsII1
.Msg = pszMsg
Select Case .SType
Case 0
'データメッセージ
Case 1
'セレクト要求
Case 2
'セレクト応答
Case 3
'セレクト解除要求
Case 4
'セレクト解除応答
Case 5
'リンクテスト要求
Case 6
'リンクテスト応答
Case 7
'リジェクト要求
Case 9
'セパレート要求
End Select
End With
-
リンクテスト応答を返信する
リンクテスト要求を受信したら、直ちにリンクテスト応答を返さなければなりません。これを送るには以下のように記述します。
.List = "LinkTestRsp"
.Reply pszMsg
.SType = 6
.Stream = 0
.Fucntion = 0
Send False
-
実行
わずか100行足らずという簡単さでHSMSクライアントの作成は完了です。お客様向けに出荷するシステムに仕上げるにはこのサンプルプログラムに機能を追加していけばいいでしょう。同じようなプログラムを一から作成することを考えると、信じられないほど簡単に作成できることが分かっていただけたと思います。
Option Explicit
Private Sub Send (bDataMessage As Boolean)
With SwingSecsII1
If bDataMessage Then
.SessionID = 0
.SType = 0
Else
.SessionID = &HFFFF
End If
.PType = 0
.EBit = False
SwingHsms1.Send .Msg
End With
End Sub
Private Sub Command1_Click(Index As Integer)
With SwingHsms1
Select Case Index
Case 0
'Connect
.Active = True
If .Active Then
SwingSecsII1.List = "SelectReq"
Send False
End If
Case 1
'Disconnect
.Active = False
Case 2
'Link test
SwingSecsII1.List = "LinkTestReq"
Send False
Case 3
'S1F1
SwingSecsII1.List = "s1f1w"
Send True
Case 4
'S1F13
SwingSecsII1.List = "s1f13w{}"
Send True
End Select
End With
End Sub
Private Sub Form_Load()
Command1_Click 0
End Sub
Private Sub SwingHsms1_Read (ByVal pszIPAddress As String, ByVal lPortNumber As Long, ByVal pszMsg As String)
With SwingSecsII1
.Msg = pszMsg
Select Case .SType
Case 0
'Data message
If (.Fucntion Mod 2) = 1 And .WBit Then
'Send default reply message
.List = "<b 0>"
.Reply pszMsg
Send True
End If
Case 1
'Select.Req
.List = "SelectRsp"
.Reply pszMsg
.SType = 2
.Stream = 0
.Fucntion = 0
Send False
Case 2
'Select.Rsp
Case 3
'Deselect.Req
.List = "DeselectRsp"
.Reply pszMsg
.SType = 4
.Stream = 0
.Fucntion = 0
Send False
Case 4
'Deselect.Rsp
Case 5
'LinkTest.Req
.List = "LinkTestRsp"
.Reply pszMsg
.SType = 6
.Stream = 0
.Fucntion = 0
Send False
Case 6
'LinkTest.Rsp
Case 7
'Reject.Req
Case 9
'Separate.Req
End Select
End With
End Sub
|
|