Savoy ActiveX Control
|
This tutorial will explain programming with Savoy by making a mini host application.
|
Specification of Mini Host
Following is the specification of mini host which will control a general wafer inspection tool.
|
Create New Project
-
Launch Visual Studio 2017 and click [New] - [Project...] from File menu.
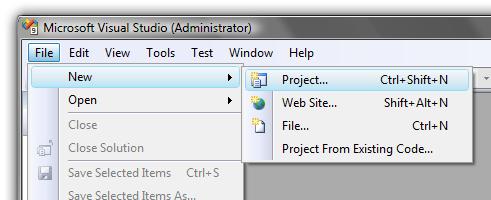
-
Choose "MFC Application" from "Visual C++" project type, and type project name and folder name.
For example, project name could be "SavoyTutorialVC2008".
If setting was OK, click "OK" button.
-
Welcome screen will appear.
Click "Next" button.
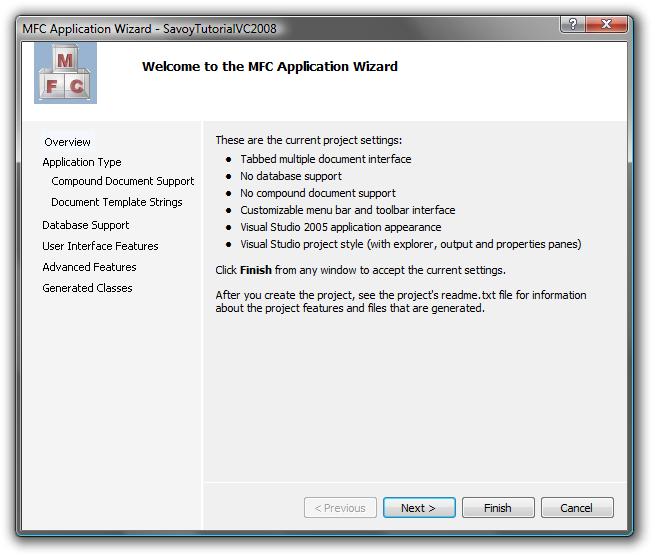
-
Choose "Dialog based" application and uncheck "Use Unicode libraries".
Since other settings are OK by
default, click "Finish" button.
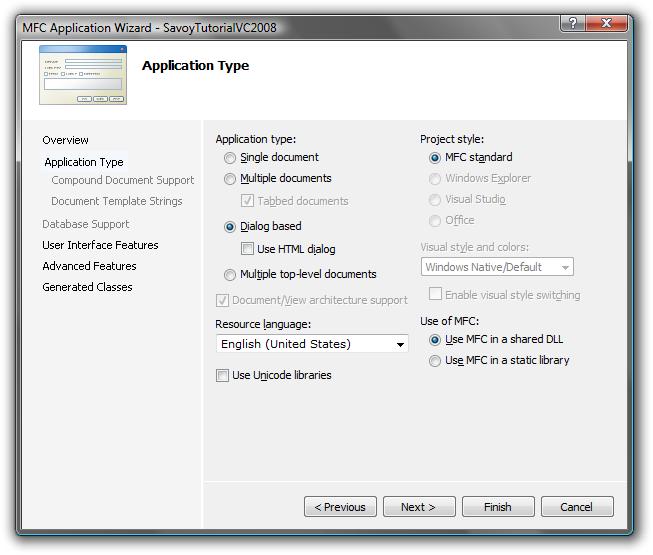
-
New project was created.
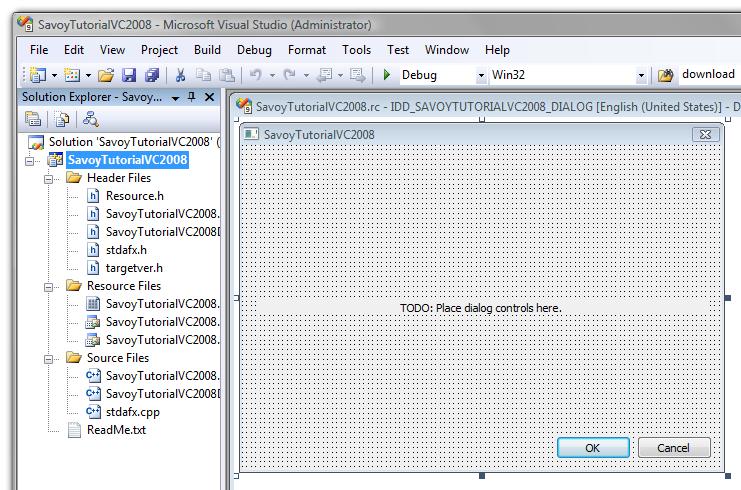
|
Add Savoy into Toolbox
|
Paste Savoy into Form
-
Place 1 SavoyHsms and 2 SavoySecsIIs on the form as follows.
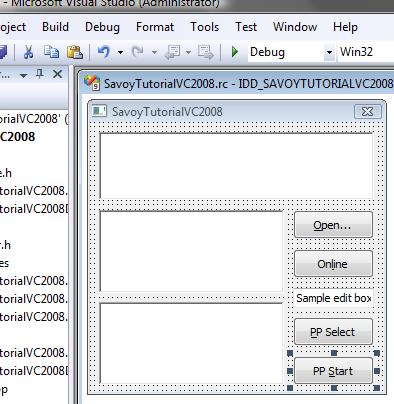
-
Assign as variable.
Right click on SavoyHsms object, and click "Add Variable" from popup menu.
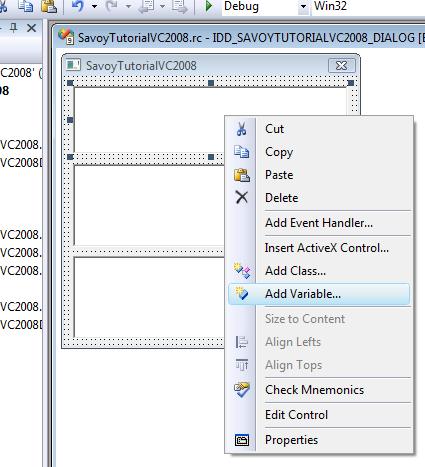
-
Change variable type to "CSavoyHsms".
The name of ".h file" and ".cpp file" will also be "SavoyHsms.h" and "SavoyHsms.cpp" respectively.
Variable name will be "m_hsms".
Click "Finish" button.
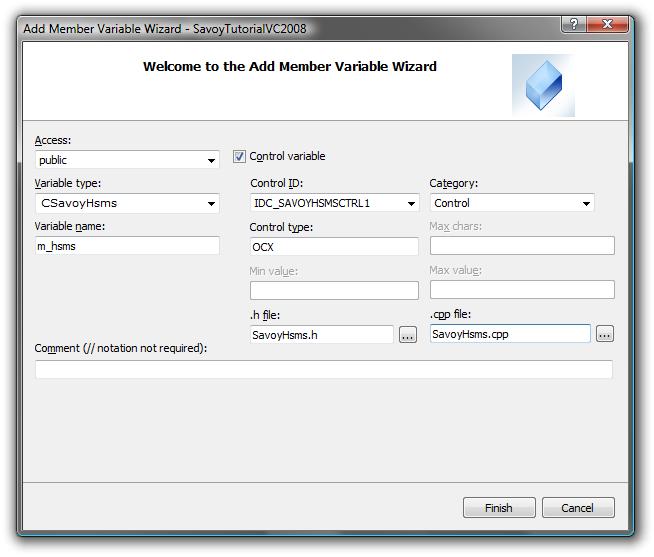
-
Do same thing for SavoySecsII control.
Change variable type to "CSavoySecsII".
The name of ".h file" and ".cpp file" will be "SavoySecsII.h" and "SavoySecsII.cpp" respectively.
Variable name will be "m_inmsg" and "m_outmsg" respectively.
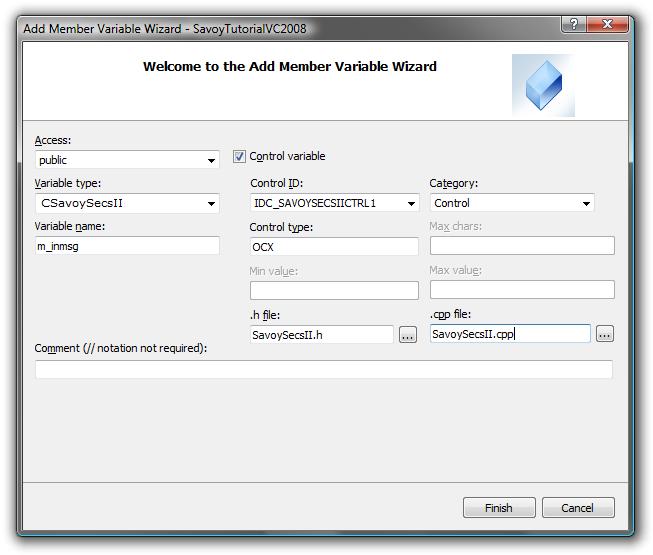
-
Variable for recipe name will be CString type and "m_strPPID".
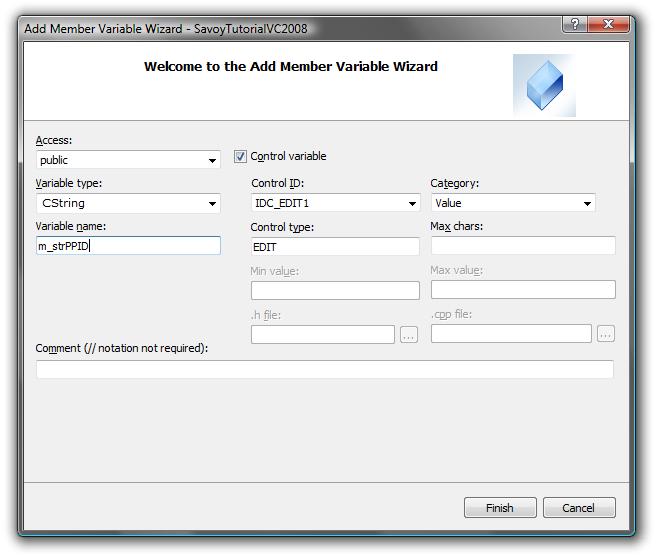
|
Overwrite Wrapper Classes
Visual C++ 2008 has some issues regarding ActiveX control wrapper class.
So Jazz Soft provided wrapper class to solve this issue.
Wrapper class could be made by Visual C++ 6.0, but "enum" is not reflected.
-
Close Visual C++ 2008 at this time.
-
Overwrite newly created "SavoyHsms.h", "SavoyHsms.cpp", "SavoySecsII.h" and "SavoySecsII.cpp" with ones provided by Jazz Soft.
-
Restart Visual C++ 2008 and reload project.
|
Process Buttons
-
Make button event handler function.
If user clicks "Open" button, communication setting dialog box of SavoyHsms will appear on the screen.
And then establish connection, if user clicks "Open" button.
Since communication setting would be saved in "Savoy.ini" file, user doesn't have to change the setting next time.
|
void CSavoyTutorialVC2008Dlg::OnBnClickedButton1()
{
// Setup
m_hsms.LoadIniFile();
if(m_hsms.Setup(""))
{
// If OK button was pressed, establish connection
m_hsms.SetConnect(true);
}
}
|
-
If user clicks "Online" button, send S1F13 message.
|
void CSavoyTutorialVC2008Dlg::OnBnClickedButton2()
{
// Send S1F13
m_outmsg.SetSml("s1f13w{}");
m_hsms.Send(m_outmsg.GetMsg());
}
|
-
If user clicks "PP Select" button, send remote command "PP-SELECT".
|
void CSavoyTutorialVC2008Dlg::OnBnClickedButton3()
{
// Send S2F41 PP-Select
UpdateData();
m_outmsg.SetSml("s2f41w{<a'PP-SELECT'>{{<a'PPID'><a'" + m_strPPID + "'>}}}");
m_hsms.Send(m_outmsg.GetMsg());
}
|
-
If user clicks "PP Start" button, send remote command "START".
|
void CSavoyTutorialVC2008Dlg::OnBnClickedButton4()
{
// Send S2F41 Start
m_outmsg.SetSml("s2f41w{<a'START'>{{}}}");
m_hsms.Send(m_outmsg.GetMsg());
}
|
|
Event Procedure
Capture events from SavoyHsms control.
Event handler function can be created from "Properties" window.
-
If Connected event occurs, send select request message.
|
void CSavoyTutorialVC2008Dlg::ConnectedSavoyhsmsctrl1(LPCTSTR lpszIPAddress, long lPortNumber)
{
// Connected
// Send select request
m_outmsg.SetSml("Select.req");
m_hsms.Send(m_outmsg.GetMsg());
}
|
-
If Received event occurs, pass incoming message to SavoySecsII control to analyze message structure.
|
void CSavoyTutorialVC2008Dlg::ReceivedSavoyhsmsctrl1(LPCTSTR lpszIPAddress, long lPortNumber, LPCTSTR lpszMsg)
{
m_inmsg.SetMsg(lpszMsg);
|
-
If incoming message requires reply message, return appropriate message such as "<b 0>".
|
switch(m_inmsg.GetSType())
{
case 0:
// Data message
if(m_inmsg.GetWbit() && m_inmsg.GetFunction()%2)
{
// Need to reply something...
m_outmsg.SetSml("<b 0>");
m_outmsg.Reply(lpszMsg);
m_hsms.Send(m_outmsg.GetMsg());
}
break;
|
-
If select request message arrives, reply select response message.
|
case 1:
// Select request
m_outmsg.SetSml("Select.rsp");
m_outmsg.Reply(lpszMsg);
m_hsms.Send(m_outmsg.GetMsg());
break;
}
}
|
|
Entire Source Code
That's it.
Since the entire source code consists of multiple files, I don't put them here.
This project was created from zero, however, the number of lines in core source code "SavoyTutorialVC2008Dlg.cpp" is only 231 lines including empty lines and comments.
Actual code we wrote was only 31 lines except comment lines.
We didn't write any configuration file or data file which typically was required by competitors' products.
Competitors' products are never as simple as Savoy.
|
|